고정 비율 그리드
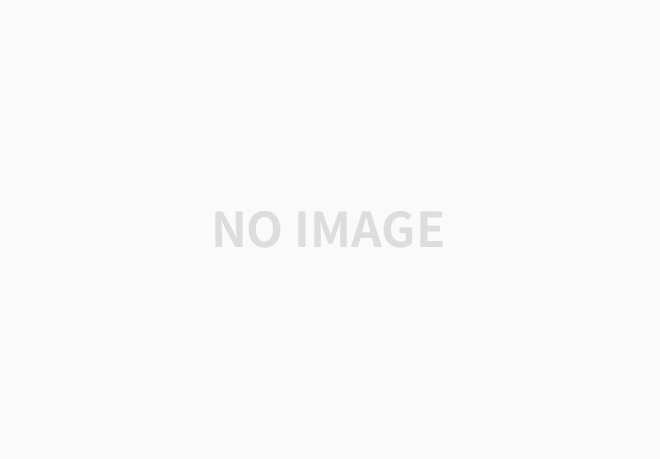
HTML
<div class="parent"> <div class="child"></div> <div class="child"></div> <div class="child"></div> <div class="child"></div> <div class="child"></div> <div class="child"></div> <div class="child"></div> <div class="child"></div> </div>
CSS
.parent { display:flex; flex-wrap: wrap; border:1px solid black; } .child { flex-basis: 20%; overflow:hidden; background-color:skyblue; box-shadow: 0 0 0 1px #000 inset; }
'개발 > 프론트' 카테고리의 다른 글
[자바스크립트] 호이스팅(Hoisting) (0) | 2020.01.30 |
---|